Eliminate Entire Class of Bugs in JavaScript with These Proven Techniques
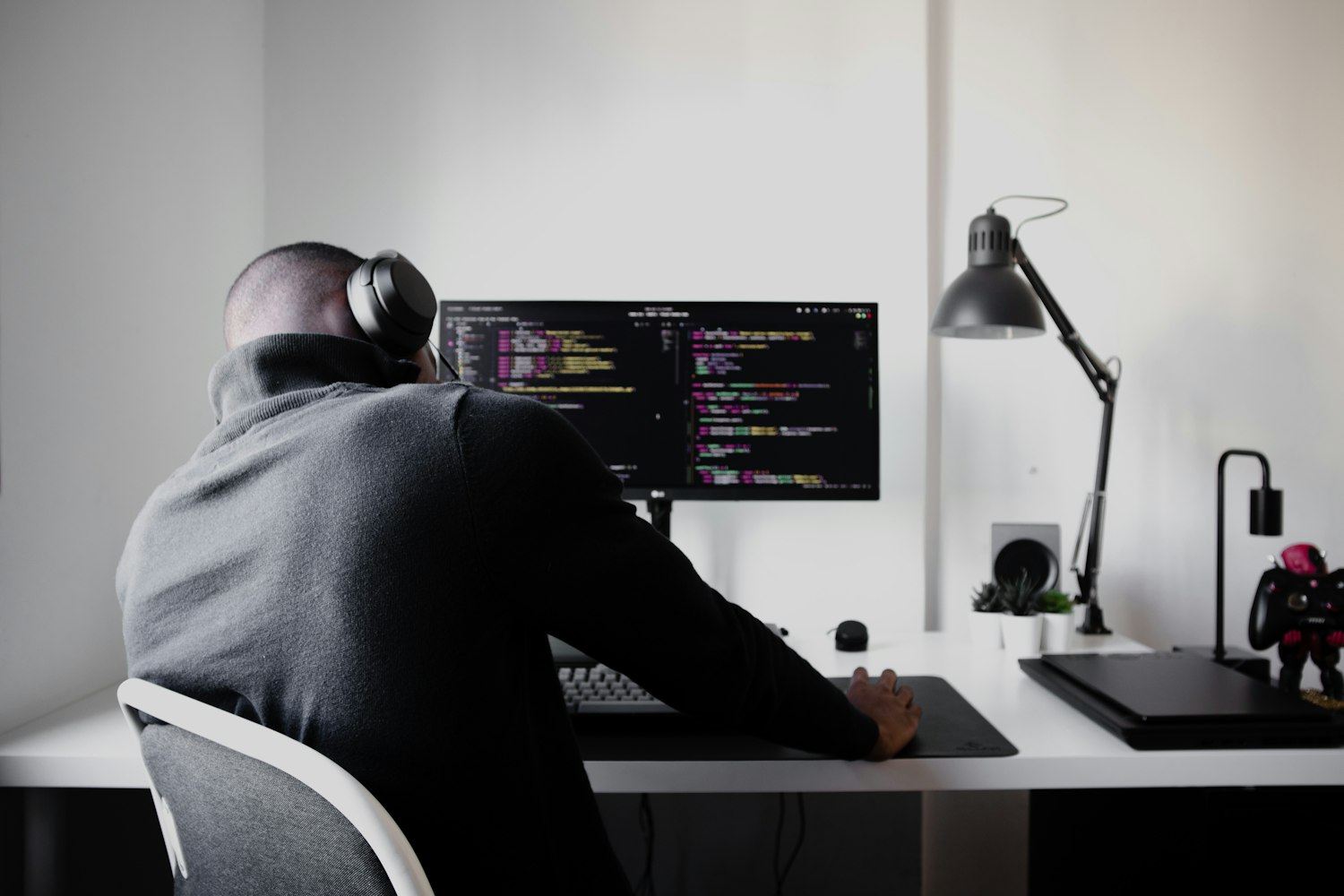
You can avoid a whole bunch of bugs headache by using some simple libraries that helps pre-analysis your code and fix it automatically saving you a lot of time looking for that missing curly bracket }
Prettier
To be short, you can write ugly code, presse CTRL+S / CMD+S and it will make your code more beautiful or prettier
Let’s say you’re afraid that the idea you have in mind runs away from you so you need to write it as quick as possible and you end up with this chunk of ugly code:
1import React from ' react'2const Button=({text, onClickHandler, colorVariant, isDisabled} )3=>{4 console.log(text)5 return (6<button style={{ backgroundColor: colorVariant }} onClick={onClickHandler}7 disabled={isDisabled}>8 {9 text}10 </button>11 )12}13export default Button;
How cool it would be if you just saved the file and it becomes this:
1import React from 'react'23const Button = ({4 text,5 onClickHandler,6 colorVariant,7 isDisabled8 }) => {9 console.log(text)10 return (11 <button12 style={{ backgroundColor: colorVariant }}13 onClick={onClickHandler}14 disabled={isDisabled}15 >16 {text}17 </button>18 )19}2021export default Button;
Well that’s Prettier.
ESLint
We all agree that javascript is such a loosy typed and dynamic language which makes it prone to developer errors, That’s why Linting became such a popular tool. So Linting is a static analysis to find problematic patterns in our code without executing it, it also helps following a language specific style guides
Eslint is a one Linting tool among others like (TSLint, JSLint, JSHint, SonarQube …)
Following our previous Prettier example :
1import React from 'react'23const Button = ({4 text,5 onClickHandler,6 colorVariant,7 isDisabled8 }) => {9 console.log(text)10 return (11 <button12 style={{ backgroundColor: colorVariant }}13 onClick={onClickHandler}14 disabled={isDisabled}15 >16 {text}17 </button>18 )19}2021export default Button;
Now as your component is ready to be shipped, you still need to make some more checks to see if you didn’t leave behind any unusual code AKA shouldn’t be there on PROD, or something that breaks your coding conventions.
in our example there is a console.log sneaking there that may leak some debugging data to prod, We also glanced a semicolon at the end of the file while in our coding conventions we decided no semicolons should be used.
on save our code will become this :
1import React from 'react'23const Button = ({4 text,5 onClickHandler,6 colorVariant,7 isDisabled8 }) => {9 return (10 <button11 style={{ backgroundColor: colorVariant }}12 onClick={onClickHandler}13 disabled={isDisabled}14 >15 {text}16 </button>17 )18}1920export default Button
As you see it kind of removed some bad parts of code and it can even add parts of code like missing parentheses, semicolons, brackets…
TypeScript
As we said before javascript is a dynamic language which means we can do some crazy things like referencing variables that don’t exist, so after our code is interpreted by the browser and it finds it broken we won’t catch it until runtime, Typescript helps us avoid this kind of errors by extending javascript with types
TL;DR, Typescript validate your javascript ahead of time with a static type checking.
Here is our javascript code test.js:
1const wasteSomeTime = () => {2 const time = new Date().getUnixTime()3 setTimeout(() => {4 console.log(time)5 }, 500)6}
let’s convert it to a Typescript code test.ts:
1const wasteSomeTime: () => void = (): void => {2 const time: number = new Date().getUnixTime()3 ~~~~~~~~~~~~~~4 setTimeout((): void => {5 console.log(time)6 }, 500)7}
As you see an error has been spotted .getUnixTime(), Isn’t this so cool ? imagine this is an object key that you’re trying to access inside an array iteration to show a list of products but the key doesn’t exist, Wouldn’t it cost you Money if users went to your website and saw such a shaming error:
1Property 'getUnixTime' does not exist on type 'Date'.ts(2339)
And it will cost you effort to debug it. But Typescript made it clear that this function doesn’t exist so you better fix it before you regret it 😄
Conclusion
What I described above is what’s called Static code analysis which is a great practice to level up the confidence of your code with less effort than writing unit tests for your entire codebase, That’s why I advice starting with this tools first then unit test what they can’t cover.